This article describes how a D1 Mini NodeMCU with a built-in ESP8266 chip can retrieve data from a website via Wifi and display it on a SPI TFT ST7735 display. This includes instructions for wiring the components and the required code that is uploaded to the D1 Mini.
Wiring the display to the D1 Mini
The first step is to connect the display to the controller. The D1 Mini is powered via the micro USB input, which can be connected to any power source. The power supply is passed to the LED and XX pin of the display via the 5V pin, and the two GND PINs are also connected to each other.
The following sketch and diagram illustrate the additional connections that need to be made to be able to control what is shown on the display.
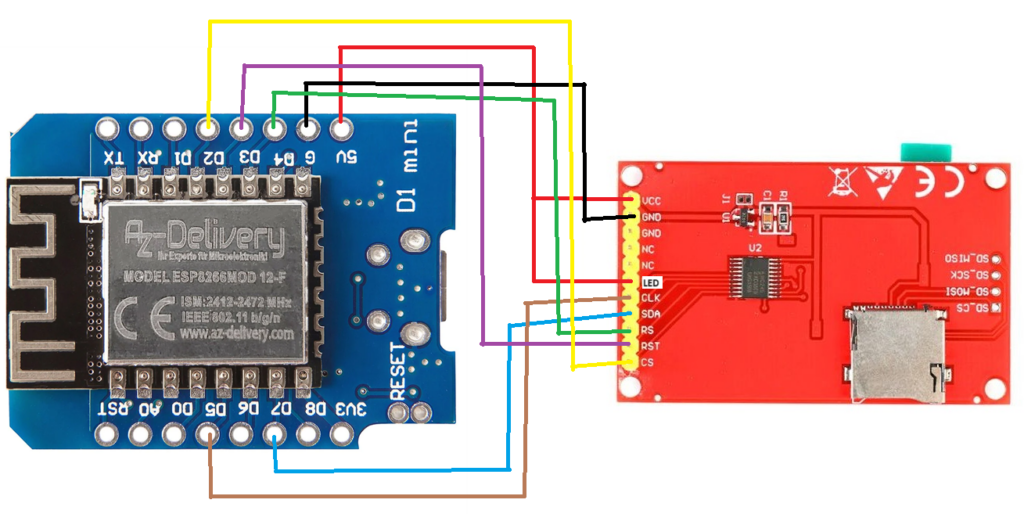
D1 Mini | TFT Display |
5V | 5V / VCC |
5V | LED |
G | GND |
D2 | CS |
D3 | RST |
D4 | RS |
D5 | SCL |
D7 | SDA |
Writing the code for the D1 Mini
The D1 Mini is programmed using the Arduino IDE. As the D1 Mini is not included in the standard installation, the following URL must be entered via “File -> Preferences -> Settings” under “Additional boards manager URLs”: https://arduino.esp8266.com/stable/package_esp8266com_index.json. It is then possible to search for “esp8266 by ESP8266 community” under “Tools -> Board -> Boards Manager” and install this manager. As soon as this has been completed, “LOLIN (WEMOS) D1 R2 & mini – esp8266” can be selected. After connecting the D1 Mini to the computer via USB cable, the port should be recognized automatically under “Tools -> Port”.
To execute the code described below, the following libraries must be installed via “Tools -> Manage Libraries”:
- Adafruit ST7735 and ST7789 Library and Adafruit GFX Library to controll the display
- ArduinoJSON to parse the response from a GET request to a JSON object
If the sketch found at the end of the article is downloaded (name of the Wifi network and the password must be adjusted in line 10 and 11!) to the D1 Mini and executed, the controller is defined as a Wifi client and a connection to the specified network is established. The establishment of the connection can be monitored via the serial monitor in the Arduino IDE. The settings “Both NL & CR” and “115200 Baud” are set for this purpose. Meanwhile, the screen is filled with a black background color and a text is displayed as a headline.
Every 30 seconds, if the network connection is maintained, a new request is executed against the API, which in this example returns some ESPN NFL data in a specific JSON format. If no error occurs, the response to the HTTP call is read and parsed into a JSON object, from which the league name and season are extracted for display.
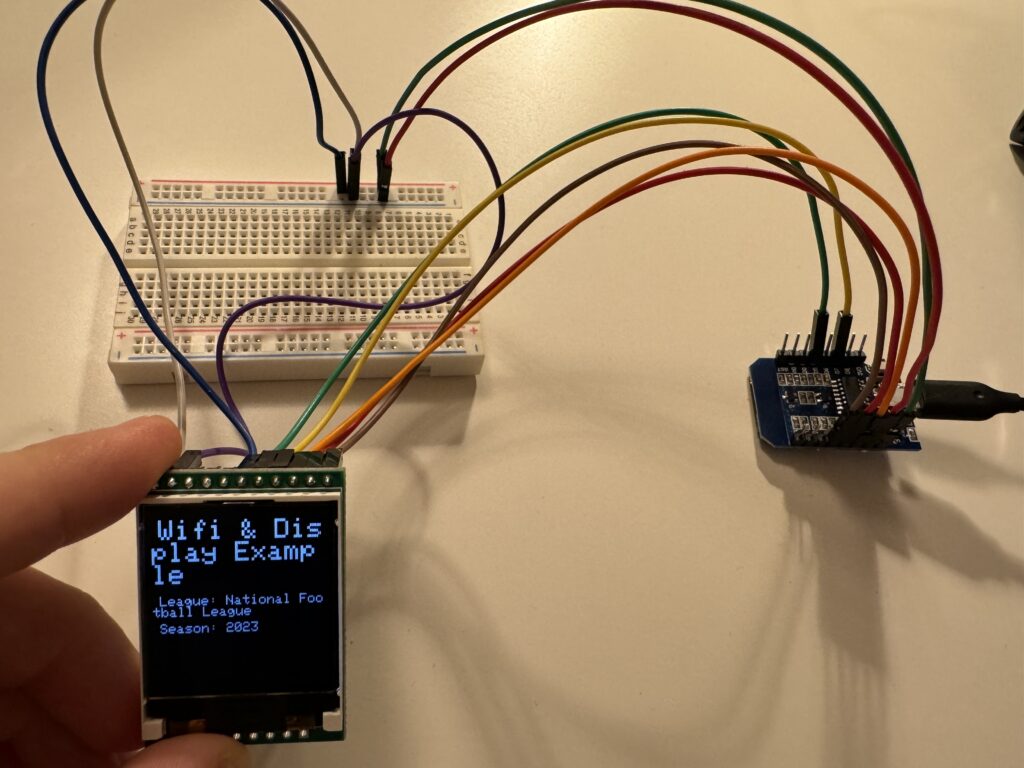
The following code was used to achieve the result shown in the picture:
#include <Adafruit_GFX.h>
#include <Adafruit_ST7735.h>
#include <SPI.h>
#include <ESP8266WiFi.h>
#include <ESP8266HTTPClient.h>
#include <WiFiClient.h>
#include <ArduinoJson.h>
#ifndef STASSID
#define STASSID "SSID"
#define STAPSK "PASSWORD"
#endif
#define TFT_CS D2
#define TFT_RST D3
#define TFT_DC D4
const char* ssid = STASSID;
const char* password = STAPSK;
Adafruit_ST7735 tft = Adafruit_ST7735(TFT_CS, TFT_DC, TFT_RST);
int delayTime = 30000;
String serverName = "http://site.api.espn.com/apis/site/v2/sports/football/nfl/scoreboard";
void setup() {
Serial.begin(115200);
tft.initR(INITR_144GREENTAB);
Serial.println("Connecting");
WiFi.mode(WIFI_STA);
WiFi.begin(ssid, password);
tft.fillScreen(ST77XX_BLACK);
tft.setTextSize(2);
tft.setCursor(5,5);
tft.setTextColor(0xFFFF);
tft.print("Wifi & Display Example");
while (WiFi.status() != WL_CONNECTED) {
Serial.print(".");
delay(500);
}
Serial.println("");
Serial.print("Connected to WiFi network with IP Address: ");
Serial.println(WiFi.localIP());
}
void loop(void) {
if (WiFi.status()== WL_CONNECTED) {
WiFiClient client;
HTTPClient http;
http.begin(client, serverName.c_str());
int httpResponseCode = http.GET();
if (httpResponseCode > 0) {
String payload = http.getString();
JsonDocument jsonPayload;
DeserializationError error = deserializeJson(jsonPayload, payload.c_str());
if (!error) {
const String league = jsonPayload["leagues"][0]["name"];
const String season = jsonPayload["leagues"][0]["season"]["year"];
showValues(league, season);
}
else
{
Serial.print("JSON Error: ");
Serial.println(payload);
}
}
else {
Serial.print("HTTP Error code: ");
Serial.println(httpResponseCode);
}
}
else {
Serial.println("WiFi Disconnected");
}
delay(delayTime);
}
void showValues(String league, String season) {
tft.drawRect(0, 85, 128, 43, ST77XX_BLACK);
tft.setTextSize(1);
tft.setTextColor(0xFFFF);
tft.setCursor(5, 60);
tft.print("League: " + league);
tft.setCursor(5, 80);
tft.print("Season: " + season);
}